General Configuration
At the Feather 32u4‘s heart is at ATmega32u4 clocked at 8 MHz and at 3.3V logic. Feather 32u4 LoRa Radio uses the extra space left over to add an RFM9x LoRa 868/915 MHz radio module. We use 868 MHz one. See complete specification here.
- Run Arduino IDE.
- You will need to install the Adafruit Package in your Arduino IDE. Copy and paste the link below into the Additional Boards Manager URLs option in the Arduino IDE preferences.
https://adafruit.github.io/arduino-board-index/package_adafruit_index.json
- Install the Adafruit boards via Tools -> Boards Manager
- You should see in menu Tools -> Board the Adafruit Feather 32u4
- Connect the Adafruit Feather using directly micro USB cable (no FTDI adapter is needed here).
- Check the appropriate COM port which is used for communication with Adafruit.
Temperature Sensor using Adafruit Feather 32u4 and DHT22
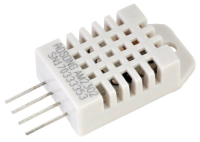
- Connect the one wire temperature sensor DHT22 to Adafruit. For data, we can use any of available digital pins, see here
- Use an addition 4.7k resistor between +3.3V and the data line.
- You need to additionally wire up the board’s IO1 pin with the board’s pin 6. You can use a simple wire bridge for that. Check the picture below to make sure you are actually using the right pins.
- Everything assembled:
- For communication with DHT22 we need Arduino library for DHT11DHT22, etc Temp & Humidity Sensors, import it to Arduino IDE
- Check if your Adafruit Feather can simply read the temperature and humidity from a sensor using Arduino IDE simple sketch:
#include "DHT.h" #define DHTPIN 2 // define data pin on Adafruit, we used 2 #define DHTTYPE DHT22 // define type of sensor DHT dht(DHTPIN, DHTTYPE); //define properties of sensor float hum; float temp; void setup() { Serial.begin(9600); dht.begin(); //initiate the communication with sensor } void loop() { hum = dht.readHumidity(); //save the value of humidity to hum variable temp = dht.readTemperature(); // save the value of temperature to temp variable Serial.print("Humidity is: "); Serial.print(hum); Serial.print("% | "); Serial.print("Temperature is: "); Serial.print(temp); Serial.println(" degrees of Celsius"); delay(1000); }
- Use serial monitor,… and it works!
- Within TheThingsNetwork https://thethingsnetwork.org/ we use the LMIC-Library https://github.com/matthijskooijman/arduino-lmic, slightly modified to run in the Arduino environment, allowing using the SX1272, SX1276 tranceivers and compatible modules (such as some HopeRF RFM9x modules).
- Import following library into ArduinoIDE: https://github.com/matthijskooijman/arduino-lmic
- Before a device can communicate via The Things Network you need to register it with an application. To use the default Over The Air Activation (OTAA) you will need to register your device with its Device EUI.
- We used adafruit-feather-32u4-lora-ota.ino sketch with slight modification.
- Change the DEVEUI, APPEUI and APPKEY.
Note: DEVEUI, APPEUI are reverse order (LSB) - Change pin mapping to:
const lmic_pinmap lmic_pins = { .nss = 8, .rxtx = LMIC_UNUSED_PIN, .rst = 4, .dio = {7, 6, LMIC_UNUSED_PIN}, };
- To send current temperature via TTN comment following:
//static mydata[] = "Hello, TTN over OTAA!";
- Don’t forget to add:
#include "DHT.h"
- Add to
void do_send(osjob_t* j)
the following:float teplota = dht.readTemperature(); dtostrf(teplota,5,2,(char*)mydata); LMIC_setTxData2(1, mydata, strlen((char*) mydata), 0); Serial.println(F("Packet queued"));
- Let LMIC compensate for an inaccurate clock. Just add this to your setup somewhere:
LMIC_setClockError(MAX_CLOCK_ERROR * 1 / 100); // Let LMIC compensate for +/- 1% clock error
This lets LMIC make the RX windows longer and start sooner so it will catch a downlink even when the clock runs up to 1% faster or slower.
- Check TTN console if payload as temperature is received: